Lua
Lua is a general-purpose CPU programming language. Pixel Composer support Lua scripting through 3 differrent nodes. Lua Global, Lua Compute, and Lua Surface.
Lua Thread
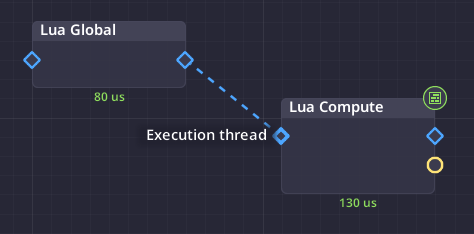
Lua nodes are connected by a thread. Node in the same thread contains the same variables. Thread also implies execution order. However, if the thread branch out to multiple nodes, the executinon order can be different in each sessions.
Lua Nodes
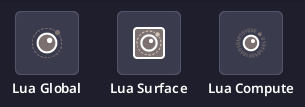
Lua Global
Lua Global is used for defining initial variable in a thread. This node won't returns any data, the only output is a lua thread that you can use in other nodes.
This node can be set to run once at the beginning, or run every frames.
Lua Compute
Compute is used to create a function that returns a value. Once the function is being created. It can be called again in other nodes with the same thread downstream.
Lua Surface
Lua Surface is used to modify a surface. All the draw* functions can only work in this node.
Syntax
Variable
You can defines a variable by simply typing the name and the value. Lua support both number and string types.
Operators
Lua also supports a similiar set of operators to other programming languange:
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Division |
% | Modulo |
^ | Exponential |
== | Equal |
~= | Not equal |
< | Less than |
> | Greater than |
<= | Less than or equal |
>= | Greater than or equal |
and | Logical and |
or | Logical or |
not | Logical not |
.. | String concatenation |
# | Length of a string |
Condition
Lua support if...then, elseif...then and else...then condition. Each block need to start with then and ends with end keyword.
if(value > 10) thenreturn 1
elseif(value < 0) then
return -1
else
return 0
end
For Loop
For loop can be created with for...do keyword. After the for keyword, you need to define the start value, the end value, and the step. The block ends with end keyword.
for i = 1, 10, 1 doprint(i)
end
While Loop
While loop can be created with while...do keyword. The block ends with end keyword.
while(value < 10) dovalue += 1
end
Repeat Loop
Repeat loop can be created with repeat...until keyword. The block ends with until keyword.
repeatvalue += 1
until(value > 10)
Functions Reference
draw(surface, x, y)
draw(surface, x, y)
drawBlend(surface, x, y, color = white, alpha = 1)
drawBlend(surface, x, y, color = white, alpha = 1)
drawTransform(surface, x, y, xs = 1, ys = 1, rot = 0)
drawTransform(surface, x, y, xs = 1, ys = 1, rot = 0)
drawGeneral(surface, x, y, xs = 1, ys = 1, rot = 0, color = white, alpha = 1)
drawGeneral(surface, x, y, xs = 1, ys = 1, rot = 0, color = white, alpha = 1)
setColor(color = white)
setColor(color = white)
setAlpha(alpha = 1)
setAlpha(alpha = 1)
setColorAlpha(color = white, alpha = 1)
setColorAlpha(color = white, alpha = 1)
getColor(x, y)
getColor(x, y)
getColorSurface(surface, x, y)
getColorSurface(surface, x, y)
drawRect(x0, y0, x1, y1)
drawRect(x0, y0, x1, y1)
drawRectOutline(x0, y0, x1, y1, thick = 1)
drawRectOutline(x0, y0, x1, y1, thick = 1)
drawCircle(x, y, radius)
drawCircle(x, y, radius)
drawCircleOutline(x, y, radius, thick = 1)
drawCircleOutline(x, y, radius, thick = 1)
drawEllipse(x0, y0, x1, y1)
drawEllipse(x0, y0, x1, y1)
drawEllipseOutline(x0, y0, x1, y1, thick = 1)
drawEllipseOutline(x0, y0, x1, y1, thick = 1)
drawLine(x0, y0, x1, y1, thick = 1)
drawLine(x0, y0, x1, y1, thick = 1)
drawLineRound(x0, y0, x1, y1, thick = 1)
drawLineRound(x0, y0, x1, y1, thick = 1)
drawPixel(x, y)
drawPixel(x, y)
colorGetRed(color)
colorGetRed(color)
colorGetGreen(color)
colorGetGreen(color)
colorGetBlue(color)
colorGetBlue(color)
colorGetHue(color)
colorGetHue(color)
colorGetSaturation(color)
colorGetSaturation(color)
colorGetValue(color)
colorGetValue(color)
getColor()
getColor()
getAlpha()
getAlpha()
colorCreateRGB(red, green, blue, normalize = false)
colorCreateRGB(red, green, blue, normalize = false)
colorCreateHSV(hue, saturation, value, normalize = false)
colorCreateHSV(hue, saturation, value, normalize = false)
colorMerge(colorFrom, colorTo, ratio)
colorMerge(colorFrom, colorTo, ratio)
setBlend(blend)
setBlend(blend)
resetBlend()
resetBlend()
randomize()
randomize()
setSeed(seed)
setSeed(seed)
random(from = 0, to = 1)
random(from = 0, to = 1)
irandom(from = 0, to = 1)
irandom(from = 0, to = 1)
abs(number)
abs(number)
round(number)
round(number)
floor(number)
floor(number)
ceil(number)
ceil(number)
max(number0, number1)
max(number0, number1)
min(number0, number1)
min(number0, number1)
clamp(number, min = 0, max = 1)
clamp(number, min = 0, max = 1)
lerp(numberFrom, numberTo, ratio)
lerp(numberFrom, numberTo, ratio)
sqr(number)
sqr(number)
sqrt(number)
sqrt(number)
power(base, exponent)
power(base, exponent)
exp(exponent)
exp(exponent)
ln(number)
ln(number)
log2(number)
log2(number)
log10(number)
log10(number)
logn(base, number)
logn(base, number)
sin(number)
sin(number)
cos(number)
cos(number)
tan(number)
tan(number)
asin(number)
asin(number)
acos(number)
acos(number)
atan(number)
atan(number)
atan2(y, x)
atan2(y, x)
dsin(number)
dsin(number)
dcos(number)
dcos(number)
dtan(number)
dtan(number)
dasin(number)
dasin(number)
dacos(number)
dacos(number)
datan(number)
datan(number)
datan2(y, x)
datan2(y, x)
rad(number)
rad(number)
deg(number)
deg(number)
dot(x0, y0, x1, y1)
dot(x0, y0, x1, y1)
stringLength(string)
stringLength(string)
stringSearch(string, searchString)
stringSearch(string, searchString)
stringCopy(string, start, length)
stringCopy(string, start, length)
stringUpper(string)
stringUpper(string)
stringLower(string)
stringLower(string)
stringReplace(string, replaceFrom, replaceTo)
stringReplace(string, replaceFrom, replaceTo)
stringReplaceAll(string, replaceFrom, replaceTo)
stringReplaceAll(string, replaceFrom, replaceTo)
stringSplit(string, delimiter)
stringSplit(string, delimiter)
print(string)
print(string)